Hey All,
So I am trying to send info into a Pay Simple API. I am not storing the data anywhere, I am simply inputting from the client to the server direct to the API request.
I have an SSL, is there anything else in particular I may need to worry about in terms of security? I figure since I am not storing the info anywhere except temporarily in the variable to make the request this will be secure enough just with SSL. Please let me know if I am mistaken.
Secondly I am trying to do the Meteor.call(‘addPaymentMethod’) and I would like to await the result from the call to determine if the number was accepted or not before continuing. What is the best way to do this? I am using the HTTP package in order to make the request.
I was trying to implement with both the async/await method as well as using the callPromise package but neither seemed to work correctly for me.
Does anyone have a quick snippet on how to await for the HTTP request to finish on a Meteor.call? Please let me know if posting my code would be helpful.
Where are you running the Meteor call from? From the client, or from another method?
Because Meteor methods run in a fiber (on the server), it will automatically await calls made using Meteor’s HTTP tool, like so:
Meteor.methods({
'addPaymentMethod': function(input) {
const result = HTTP.post('https://api.somepaymentprovider.com/paymentMethods/create', {
data: input,
headers: { key: process.env['PAYMENT_API_KEY'] },
});
// don't need to use a callback, promise or async/await to wait for async http call to complete
if (result.error) {
throw new Meteor.Error('Could not create payment method, reason: ' + result.error);
}
return result
},
});
2 Likes
This is a pattern we use in certain cases, might be useful for you.
(a) Client side async call to add a new card (new source) to stripe. In the case of stripe, they give you a widget, which is where the underlying source
object comes from. The handleSource is triggered from the stripe widget.
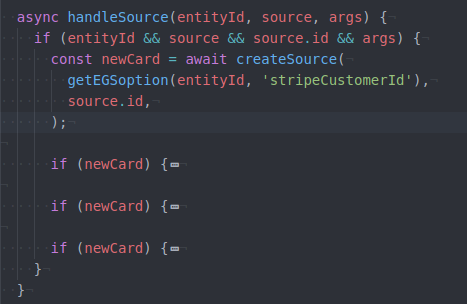
(b) Client side middleware function to promisify. Note, not strictly necessary, but the pattern is useful in certain ways. Generally, we could do other stuff here, but which we do not necessarily want on server.
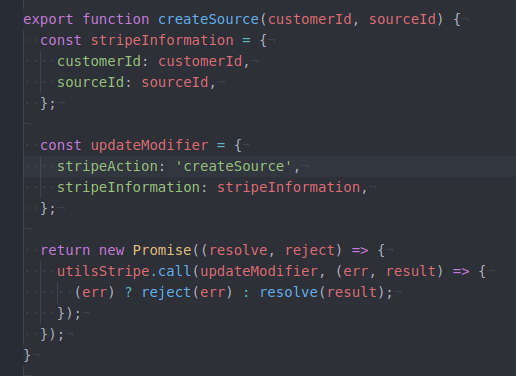
(c) Method in server
If it looks like overkill in this specific case, it may well be. But the pattern is useful in a number of ways.
Note: Am self taught - if some huge mistake, please tell me. NOT a professional programmer.
1 Like
One thing I can see that you could improve is to initialize the stripe instance outside the method and re-use it for each call, instead of creating a new one each time.
Otherwise, looks great!
Using an async function in the Meteor method is particularly useful because the stripe SDK is already promisified, and you can await those promises
1 Like
Thanks, That certainly did the trick! I was trying to use the call back on the HTTP on server side so I guess that was messing it up. I must say you guys are all super helpful I am very grateful for this community.