I have the following collection:
{
"scheduled_date" : "09.06.2015",
"scheduled_time" : "20:00",
"scheduled_weekday" : "Ti",
}
{
"scheduled_date" : "09.06.2015",
"scheduled_time" : "21:00",
"scheduled_weekday" : "Ti",
}
{
"scheduled_date" : "09.06.2015",
"scheduled_time" : "22:00",
"scheduled_weekday" : "Ti",
}
{
"scheduled_date" : "16.06.2015",
"scheduled_time" : "20:00",
"scheduled_weekday" : "Ti",
}
{
"scheduled_date" : "16.06.2015",
"scheduled_time" : "21:00",
"scheduled_weekday" : "Ti",
}
{
"scheduled_date" : "16.06.2015",
"scheduled_time" : "22:00",
"scheduled_weekday" : "Ti",
}
{
"scheduled_date" : "23.06.2015",
"scheduled_time" : "20:00",
"scheduled_weekday" : "Ti",
}
{
"scheduled_date" : "23.06.2015",
"scheduled_time" : "21:00",
"scheduled_weekday" : "Ti",
}
{
"scheduled_date" : "23.06.2015",
"scheduled_time" : "22:00",
"scheduled_weekday" : "Ti",
}
And I would like to find the most efficient way to populate the following table? Where the th row represents the grouped dates and td row the data which scheduled date is within the th.
Hey I’m not totally clear (from the code example) as to what you are trying to do. Could you give me a little more info?
@ryanswapp Hello I updated what I am trying to do.
Currently I am doing this in way that I run two helpers and each loops. Second each loop is ran inside the first one
1st helper is getting all the dates from the collection, then using underscore.js to populate the list of unique dates and finally pushing the list to the #each loop which generates th-elements for the table.
2nd helper and each loop is working inside the first one using the scheduled date in the th-element as a filter
html
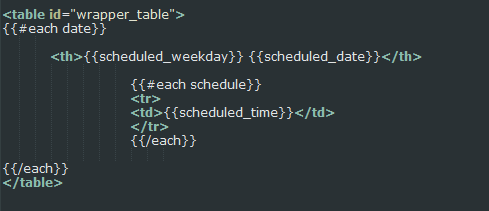
javascript
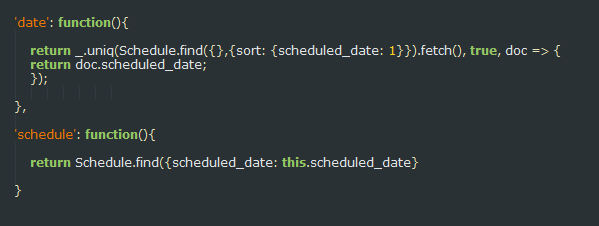
Instead of making multiple find()
calls, you could consider loading everything once, converting it into an object that represents your table, storing the data in a ReactiveVar, then leveraging helpers for the th/td’s specifically. Something like:
main.js:
...
Template.body.onCreated(function onCreated() {
this.scheduleData = new ReactiveVar({});
const data = {};
Collection.find().forEach((item) => {
const key = `${item.scheduled_weekday} ${item.scheduled_date}`;
if (data.hasOwnProperty(key)) {
data[key].push(item.scheduled_time);
} else {
data[key] = [item.scheduled_time];
}
});
this.scheduleData.set(data);
});
Template.body.helpers({
dates() {
return Object.keys(Template.instance().scheduleData.get());
},
schedule(date) {
return Template.instance().scheduleData.get()[date];
}
});
...
main.html:
...
<table>
{{#each dates}}
<tr><th>{{this}}</th></tr>
{{#each schedule this}}
<tr><td>{{this}}</td></tr>
{{/each}}
{{/each}}
</table>
...
Regarding to the “loading everything at once”, would the global or session variable do the same thing as ReactiveVar in this particular case?
Yes you could use Session instead (just be aware of the pros/cons).