As the title suggest I want to send data, collection in this case, to the client side through and then display it.
So what I’ve done is creating a publications file that’s imported into the server in main.js, it grabs the collection I was the client side to receive as publishes it.
import Links from '../collections/links.js';
Meteor.publish('links', function(){
return Links.find({});
});
and later on into my template file where I want everything to be display I import and but I get an error in the console telling me that Links
isn’t global
import './home.html'
import { Template } from 'meteor/templating'
Template.home.onCreated(function() {
var self = this;
self.autorun(function() {
self.subscribe("links");
});
});
Template.home.helpers({
'displayLinks'(){
return Links.find();
}
});
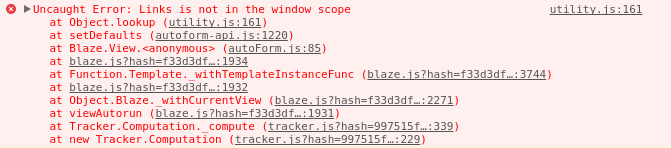
Oh and I try to display the data by typing the following line in the HTML file
{{#each displayLinks}}
{{> link}}
{{/each}}
Can we see your folder structure and the contents of links.js
, please?
Can you access the folders here or you’re blocked?
https://ide.c9.io/harry97/flame
It’s asking for a login - so blocked.
links.js
import SimpleSchema from 'simpl-schema';
import { Tracker } from 'meteor/tracker';
// Required AutoForm setup
SimpleSchema.extendOptions(['autoform']);
SimpleSchema.debug = true;
Links = new Mongo.Collection("links");
LinksSchema = new SimpleSchema({
url: {
type: String,
label: 'Input',
regEx: SimpleSchema.RegEx.Url,
index: true,
unique: true,
autoform: {
afFieldInput: {
type: "url",
placeholder: "Place your links here...",
}
}
},
encodedUrl: {
type: String,
optional: true,
autoValue: function() {
var url = this.field("url");
if (url.isSet) {
return Meteor.call("getBitlyUrl", url.value );
} else {
this.unset();
}
}
}
}, { tracker: Tracker });
Links.attachSchema(LinksSchema);
I hope this suffice, if not please notify me, thanks!
Given that you’re using import/export
for mudules you should really use export const Links = new Mongo.Collection("links");
. Also, you said this in your OP:
a publications file that’s imported into the server in main.js
However, the only main.js
I see in your folder structure is under client/
, not server/
.
You basically need to import { Links } from '/imports/api/collections/links';
in your server code and in your client code. The server part is necessary to ensure that a physical MongoDB collection is created for you. The client part is to ensure your pub/sub delivers data from server to client.
You don’t actually need the autorun
in your onCreated
- that’s only needed if your subscription references reactive variables in its parameter list for the publication. It won’t do any “harm” - it’s just unnecessary.
I did as you suggest (exporting Links constant) but the error persisted, as for the main.js, if you actually expanded the image, you’d find another main.js in server folder. I don’t just import everything directly into it, I import it into the startup/server/index.js file then import that index.js into the main one.
I’ve pushed everything into this repo, so you can view it comfortably since I struggle to explain myself - https://github.com/Harry97/meteor-url-shortener
Just type in npm start
and everything shall fire up correctly