pipeline = [{
$group: {
'_id': this.userId,
'hours': {
$sum: '$hours'
},
'magazines': {
$sum: '$magazines'
},
'brochures': {
$sum: '$brochures'
},
'books': {
$sum: 'books'
}
}
}, {
$project: {
userId: '$_id',
hours: '$hours',
magazines: '$magazines',
brochures: '$brochures',
books: '$books'
}
}];
var aggregateResult = Reports.aggregate(pipeline);
the above gets published and subscribed into client alright, and shows as in client console:
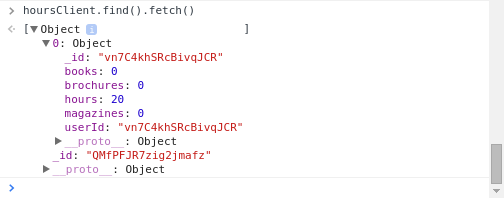
How can I access the, say, hours
field in template?
With find().fetch()
you get an array of objects, which means that you would typically write a helper:
Template.showAggregates.helpers({
groups() {
return hoursClient.find().fetch(); // or return hoursClient.find();
}
});
and then in your template:
<template name="showAggregates">
<ul>
{{#each group in groups}}
<li>{{group.userId}}, {{group.books}}, {{group.brochures}} ... </li>
{{/each}}
</ul>
</template>
You can also omit the fetch()
- just return a cursor. Normally, cursors help limit reactive changes in a template. In the case of an aggregation result, I suspect that it will make no difference.
Finally, note that I have used ES2015 syntax for the helper, and followed the new guide recommendations to use each ... in
, rather than just each
.
I’ve rigged the hoursClient collection so that, it’ll always contain only a single row (coming from sql, need to get my terms right in mongodb).
Thus, in console in client, I could do this:
x = hoursClient.find().fetch()
x[0][0].hours
Maybe findOne is what I need?
In that case you could rig your helper with findOne
as you suggest:
Template.showAggregates.helpers({
group() {
return hoursClient.findOne();
}
});
and your template:
<template name="showAggregates">
<div>{{group.userId}}, {{group.books}}, {{group.brochures}} ... </div>
</template>