Thanks waldgeist for your answer.
Yes, the onClick() handler was just for demonstration. I also guessed Blaze handlers were destroyed during jquery DOM manipulation APIs, but I was not sure if it was just technically impossible or a Blaze bug?
I did tried Blaze conditional tags. In my first attempt, injecting uncompleted/partial html fragment by Blaze conditional tags didn’t work:
{{#if stylingBegin}}
<div style='bla'> <!-- open div -->
{{/if}}
<btn1/>
<btn2/>
{{#if stylingEnd}}
</div> <!-- close div -->
{{/if}}
<btn3/>
While it makes sense that jQuery DOM manipulating APIs should not allow uncompleted DOM nodes to be inserted into doc, I think it’s kind of pity that Blaze couldn’t handle the above. I guess Blaze could have a staging area to store the doc fragment related to the template being processed, and a template can be seen as a closure for that doc fragment where the confirmation that the doc fragment is completed or not can be made in the final processing step for that template.
For this example it can still work like this:
{{#if styling}}
<div style='bla'> <!-- open div -->
<btn1/>
<btn2/>
</div> <!-- close div -->
{{else}}
<btn1/>
<btn2/>
{{/if}}
<btn3/>
… where I may further sub-template the repeated block <btn1/> <btn2/>
to make it more manageable. The same attempt however does not work for the following case, which is the real case that I am on:
I defined the following template:
{{#each ListItems}}
{{name}}
{{/each}}
to display a collection named ‘ListItems’ in this format:
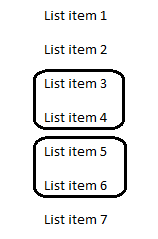
In the desired view, certain adjacent items should be displayed in a box. I have the condition for the first item to be in the first box, the last item to be in the first box, the first item to be in the second box, the last item to be in the second box, and so on, where the number of items in boxes vary, and boxes are mixed with no-boxed items in the view as showed.
The following did not work for me because the problem of uncompleted html fragment mentioned above:
{{#each listItems}}
{{#if boxStart}}
<div class='well'>
{{/if}}
{{name}}
{{#if boxEnd}}
</div>
{{/if}}
{{/each}}
My best try so far is:
{{#each listItems}}
<div id='{{boxStart}}'></div>
{{name}}
<div class='{{boxEnd}}'></div>
{{/each}}
{{deferStyling}}
Template.myTemplate.helpers({
boxStart(){
if ( someCond ){
return "box-start-"+this._id;
} else {
return "";
}
},
boxEnd(){
if ( someCond ) {
return "box-stop";
} else {
return "";
}
},
deferStyling(){
$('[id^="box-start-"]').each(function(idx){
$(this).nextUntil(".box-stop").wrapAll("<div class='well'/>");
}
},
});
I now got the view, but the events created for the items that are displayed inside the boxes do not work any more as showed in the first example.
What did I miss along the way? Is there a different trick to achieve this?